Record a packet in gqrx using the I/Q Recorder (ctrl+I), making sure not to use too high sample rate (1Msps worked fine for me).
Open I/Q recording in inspectrum, making sure to select the correct sample rate.
Right click → Add derived plot → Frequency plot, center on UKHASnet packet.
Enable time selection cursors, select one time unit of the preamble. Increase symbol number, and make sure the lines align correctly with the symbols. Repeat this for the whole packet.
Right click frequency plot → extract symbols. You'll get a list of floats on the command line.
Copy the symbols into the list x in the python script below, and run the script. The included example outputs the following:
{'checksum': '\xde\xc2', 'length': 34, 'data': '9xV3.23,3.69,3.91T22.7Z0R-33[OSr1]'}
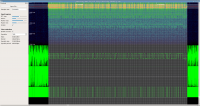
#!/usr/bin/env python
# -*- coding: utf8 -*-
# pip install --user bitstring
import bitstring
"""
* Preamble of at least three bytes of 0xAA.
* Two synchronisation bytes: 0x2D 0xAA.
* A single length byte between 0 and 64 (0x00 and 0x40).
* The number of data bytes as described by the length byte, in the
format described in the Layer 3 section below.
* Two checksum bytes, calculated as described below.
"""
# Raw input (inspectrum frequency plot extracted symbols)
x = [0.216841, -0.261636, 0.219096, -0.266524, 0.212065, -0.260867,
0.221935, -0.249724, 0.221802, -0.260077, 0.220824, -0.260648, 0.210396,
-0.264013, 0.215528, -0.253642, 0.233618, -0.258195, 0.219111,
-0.265372, 0.214256, -0.267808, 0.209937, -0.25991, 0.232465, -0.25041,
-0.258406, 0.217401, -0.263702, 0.218179, 0.211704, -0.268159, 0.229335,
0.230407, -0.253414, 0.217899, -0.25817, 0.218009, -0.267789, 0.215056,
-0.254367, -0.246849, -0.249974, 0.222519, -0.260761, -0.26241,
-0.261301, 0.218838, -0.25504, -0.249699, -0.248335, 0.235878, 0.218087,
0.217552, -0.259925, -0.260829, 0.219753, -0.253281, 0.22945, 0.232853,
0.214607, 0.217179, -0.263678, -0.261544, -0.268515, -0.267577,
0.225176, -0.250258, 0.231237, -0.265923, 0.214075, 0.214163, -0.260433,
-0.26759, -0.262872, 0.224049, 0.226019, -0.264601, -0.264295, 0.217848,
0.216708, -0.267425, -0.264499, 0.220222, -0.25903, 0.220861, 0.217432,
0.213846, -0.266552, -0.265628, -0.26728, 0.218866, 0.223372, -0.252216,
-0.257527, 0.218207, -0.26554, -0.266194, -0.263852, 0.214561, 0.222652,
-0.255715, -0.258746, 0.216042, 0.214921, -0.262316, -0.265843,
0.213283, -0.262132, 0.234911, 0.222425, -0.258964, -0.268212,
-0.261948, -0.261823, 0.211988, 0.218162, -0.262929, -0.248741,
0.225102, 0.219269, -0.266022, -0.265968, 0.217225, -0.267007, 0.217196,
0.235238, 0.227095, -0.25925, -0.266509, -0.262533, 0.215291, 0.216175,
-0.270646, 0.227622, 0.232801, -0.249791, -0.247158, -0.266351, 0.21378,
0.218169, 0.214519, -0.25927, -0.251352, 0.232727, -0.251546, -0.272696,
0.210396, -0.264262, 0.212666, 0.22186, -0.260639, -0.257088, -0.253097,
-0.259826, 0.215589, 0.216688, -0.265696, -0.269624, 0.220937, 0.217404,
-0.255146, -0.249656, 0.220375, -0.267294, 0.218417, 0.213958, 0.215574,
-0.264102, -0.262655, -0.253592, 0.219836, 0.220085, 0.221188,
-0.261837, -0.260646, 0.214605, -0.264604, -0.267797, 0.229365,
0.237114, -0.260418, -0.266878, -0.263119, 0.21506, -0.263434, 0.213958,
-0.250937, 0.227602, -0.24999, 0.223627, -0.264232, -0.259437,
-0.255263, -0.264628, 0.225421, 0.231303, -0.24944, -0.249487, 0.21535,
-0.26258, -0.262921, -0.259475, 0.223044, 0.227399, -0.24976, -0.246236,
0.218301, -0.260648, -0.26285, -0.261321, 0.217338, -0.256522, 0.226121,
0.234016, 0.231818, -0.262323, -0.264795, -0.266239, 0.221945, 0.217747,
-0.260885, 0.224342, 0.228173, 0.217476, -0.264719, 0.219541, -0.262284,
0.212248, 0.21203, -0.264602, 0.225842, -0.261688, -0.26651, -0.262665,
0.223527, 0.221421, -0.262174, -0.263653, -0.260515, -0.25002,
-0.254465, 0.219979, -0.26266, 0.216723, -0.262255, -0.264995, 0.217492,
-0.245421, -0.249774, -0.252032, 0.221862, -0.257836, 0.220338,
0.220344, -0.26288, 0.232661, -0.250644, -0.246684, 0.224459, 0.215851,
-0.258179, -0.259633, 0.218951, 0.218075, -0.245128, -0.253764,
0.228257, 0.22736, -0.266995, -0.26488, 0.216476, 0.216074, -0.261651,
0.222593, -0.253993, 0.23501, 0.218582, -0.260957, 0.217979, 0.219902,
-0.263794, 0.223031, -0.255528, -0.245308, 0.221199, 0.219862, 0.219594,
0.221592, -0.266644, 0.22015, -0.261503, 0.222219, -0.249429, -0.253903,
0.220405, 0.218208, -0.258208, 0.213865, 0.215591, 0.218854, -0.243035,
-0.24893, 0.225554, -0.256934, -0.260774, -0.255451, 0.216236, 0.211297,
-0.248017, -0.24797, -0.248842, 0.227713, -0.25972, 0.219987, -0.263378,
0.217475, 0.217265, 0.227285, -0.249343, 0.234129, 0.235813, 0.21725,
-0.26081, 0.218495, 0.219381, 0.218673, 0.225591, -0.250235, 0.227448,
0.222044, -0.2629, -0.269487, -0.266359, -0.260572, 0.216512, -0.337241,
-0.260326, -0.265867, -0.265165]
# Binary threshold more or less than 0
y = [1 if n>0 else 0 for n in x]
# Create BitArray of our data
z = bitstring.BitArray(bin=''.join([str(n) for n in y]))
# Pad bitstream for 8-bit bytes
while len(z) % 8:
z.prepend('0b0')
# Discard preamble and align bytes to sync bits
z <<= z.find('0x2daa')[0]
# Discard sync bits
z <<= 16
# Get packet length
l = ord(z.bytes[0])
z <<= 8
# Get packet content
data = z.bytes[:l]
z <<= 8*l
# Get checksum
cs = z.bytes[:2]
packet = {
'length': l,
'data': data,
'checksum': cs
}
print(packet)